
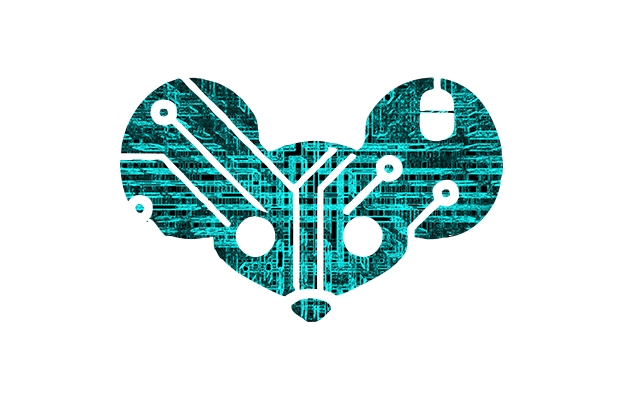
I can think of ways you could please me 🚀🤤
I can think of ways you could please me 🚀🤤
What an effortless Troll
The client works fine, it’s just the virtual file system option that turns off after a reboot.
Yes, but I can’t get the virtual file system/on-demand sync to work properly. It turn off every time I reboot. I gave up after a while since it’s experimental for now anyway.
Thanks! I looked at it but I would like to stop giving money to MS. So my plan is to ultimately move away from OneDrive.
Thank you! I thought was going nuts. It’s been such a long time since I had think about whether something is synced or a way to set it up. With OneDrive I could just grab the files I needed from the cloud and push them off the drive if I needed space. It really took away any hassle about sync.
It really feels like moving to Linux is a step back 10 years when it comes to cloud storage.
I also tried nextcloud but the smart / on-demand/ virtual file system is experimental in the Linux client and doesn’t work as seemlesly as OneDrive. Besides being turned off every time I restart.
Funnily, or sadly enough, OneDrive integration is one of the things I miss from my windows days. It’s just extremely convenient how it’s integrated into explorer and office. And how well the smart/ on-demand sync works. I can’t find a setup to replicate this on Linux.
That being said I don’t intend to go back and this move is insane.
Yes, contradicting the claim that it’s “more objective”.
I was pretty desperate for a job at that point and didn’t even tell him what a fucking idiot he is.
But it’s all good, I landed another job with roughly 60% higher pay. Two interviews, one 20min for screening, and a second one 1h with higher ups. They did ask me to prepare and present a case to them for the second interview. But I was fine with that, I could tell they are serious.
Applied for a job with a 6 tier interview process for an entry level support position. Tier 2 was a 45(!!!)min assessment test. Queue level 3 were we spend 20min discussing the results. The 20min end with the interviewer telling me they filled the position the day before but he wanted to give me the courtesy of a talk about the test anyway. What . The . Fuck. You wasted both of our time?!
Lol Jesus would hate that, remember when he went to the Pharisees and went like
You guys fucking slay, LOVE IT
Moon wen? 🚀🚀🚀
You can call me ignorant but the bible ain’t lying son
Lol everyone knows it doesn’t exist. Or where is it mentioned in the bible?! That’s right, nowhere
Depends on how you do it I suppose. If you use the print to pdf dialog f.ex. it would.
Excellent, I’ll have a look at it. Thank you!
It’s a shitty Synology Nas with extreme slow speeds. That’s only amplified by slow upload speeds when I’m not in my local network.
The main difference, and that’s also the difference to the way Gnome handles cloud storage like GDrive, is that I don’t want a network storage. I want integration into my file system, and I want automatic upload of what I’m working on. And I want the ability to say: this directory needs to be available offline. Without having to copy it from my nas to local storage and back again.
Google has a deal with reddit as well. https://www.reuters.com/technology/reddit-ai-content-licensing-deal-with-google-sources-say-2024-02-22/?utm_source=reddit.com
But I don’t think it’s just an issue with the dataset. It’s the false promise of these LLMs having a fucking clue what a good search result is and what is not. They don’t. They are just good at creating text that sounds plausible. That’s not what searching for factually correct information is about though.
Geminis answer: LLMs cannot perform the computations needed to find a SHA-512 hash with specific characteristics. This requires specialized hardware and software designed for brute-force calculations.
ChatGPT: Yes, getting an AI to solve a problem like finding a SHA-512 hash with 12 leading zeros would indeed take a significant amount of computational time and resources. This is because it would require brute-forcing through a vast number of inputs to find one that produces the desired hash, which is a computationally intensive task.
Finding a SHA-512 hash with 12 leading zeros involves iterating through possible inputs and hashing each one until you find a hash that starts with 12 zeros. This is a classic example of a proof-of-work problem, similar to what is used in cryptocurrency mining.
Here’s a Python script that demonstrates how one might go about attempting this task:
import hashlib def find_hash_with_leading_zeros(target_zeros): target = '0' * target_zeros nonce = 0 while True: input_str = f'{nonce}' hash_result = hashlib.sha512(input_str.encode()).hexdigest() if hash_result.startswith(target): return nonce, hash_result nonce += 1 # Example: Find a SHA-512 hash with 12 leading zeros target_zeros = 12 nonce, hash_result = find_hash_with_leading_zeros(target_zeros) print(f'Nonce: {nonce}') print(f'Hash: {hash_result}')
Running this script can take a very long time, especially as the number of leading zeros increases. The number of possible combinations grows exponentially, making it a time-consuming process.
To actually implement this in a controlled and efficient manner, specialized hardware (like ASIC miners used in cryptocurrency mining) would be required. This is beyond the capabilities of typical general-purpose CPUs and would indeed tie up computational resources for a significant amount of time.